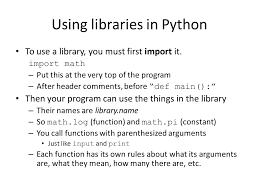
For using math functions we have import the math module which contains all the mathematical functions like sin,cos.. etc.
FOR IMPORTING MATH MODULE;
USE THE CODE:
import math
some imprtant math functions in python
1)
math.
ceil
(x)
Return the ceiling of x as a float, the smallest integer value greater than or equal to x.
example:
math.ceil(4.1) returns 5
2)
math.
floor
(x)
Return the floor of x as a float, the largest integer value less than or equal to x.
example:
math.floor(4.9) returns 4
3)
math.
factorial
(x)
example:
math.factorial(3) returns 6
4)
math.
log
(x[, base])
With one argument, return the natural logarithm of x (to base e).
With two arguments, return the logarithm of x to the given base,calculated as
log(x)/log(base)
.
5)
math.
pow
(x, y)
Return
x
raised to the power y
. Exceptional cases follow Annex ‘F’ of the C99 standard as far as possible. In particular, pow(1.0, x)
and pow(x, 0.0)
always return 1.0
, even when x
is a zero or a NaN. I6)math.
sqrt
(x)- Return the square root of x.
- 7)
math.
sin
(x) - Return the sine of x radians.
8)math.
tan
(x)- Return the tangent of x radians.
- 9)
math.
pi
- The mathematical constant π = 3.141592…, to available precision.
10)math.
e
- The mathematical constant e = 2.718281…, to available precision.
Comments
Post a Comment